Swift, iOS & OS X Programming
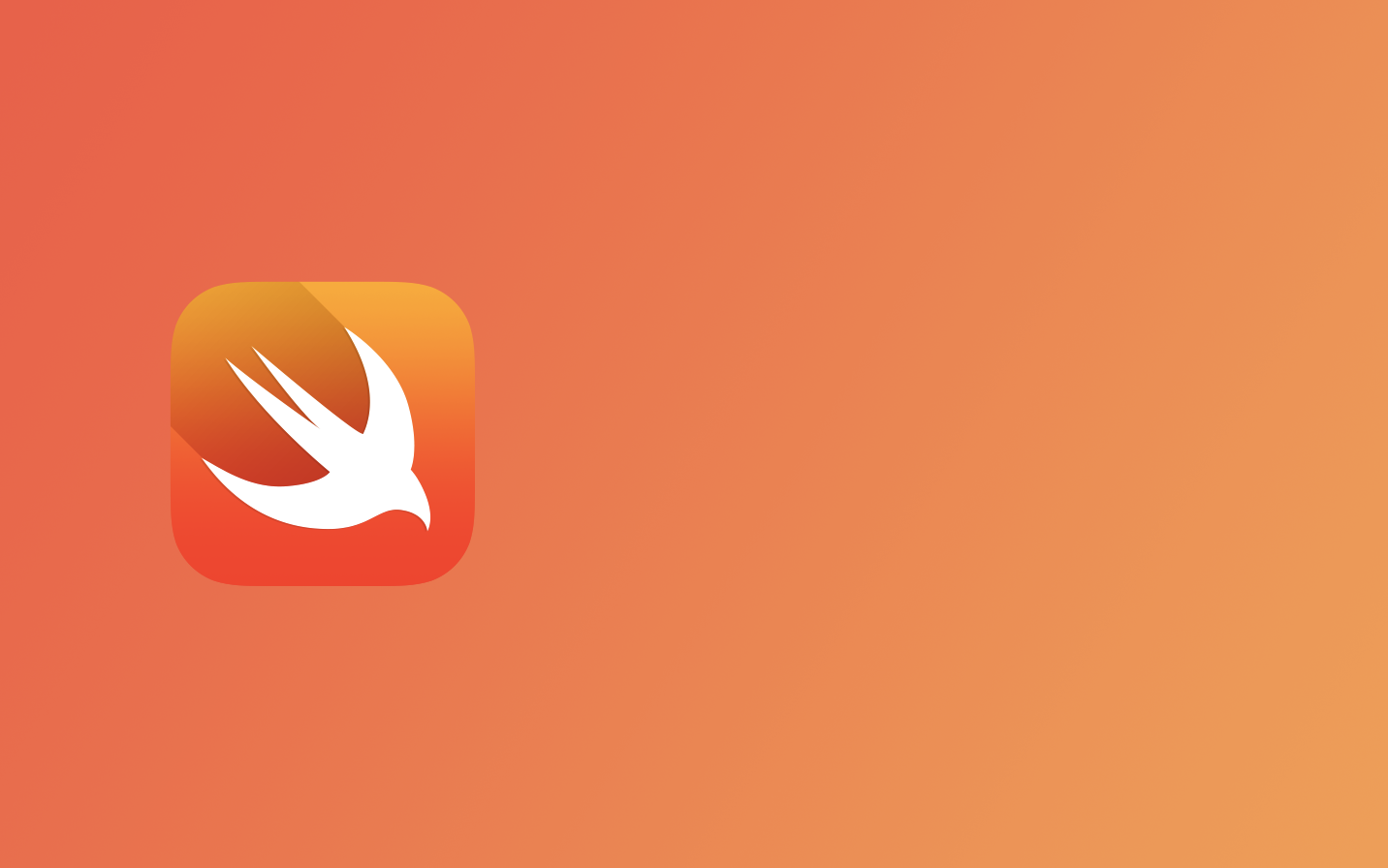
For years I put off learning how to make iOS apps. Ever since their introduction, back when iOS was called iPhone OS, I wanted to craft something but Objective-C and its obscurity put me off. The syntax was ugly and the naming conventions weren't what I was used to.
For example, methods in Objective-C don't exist. Well, I guess they do but they're called messages. To get an object to do something for you, you send it a message. It's strange for someone coming from a web background, knowing only JavaScript, PHP and a little Ruby.
Because I already knew HTML, CSS, and JS, whenever a project came in for a mobile app I would opt to just build a "hybrid" app. That is to say one that utilises web technologies and can run on both iOS and Android. For a long time this involved a lot of leg-work to make the app look and feel like it even remotely belonged to the respective platform. You'd have to style everything up yourself and then just bung it in a Cordova/PhoneGap/Titanium wrapper.
Ionic came along and fixed a lot of that. Bringing with it a solid framework, set of styles, and boilerplate interfaces to make development rapid. The problem with it came when you wanted to access some of the device's native APIs. Sure there's ngCordova which covers a fair amount of them but there's still stuff missing like MapKit. Oh, and now the Apple Watch is here, how do you even go about building something for that with HTML5?
Objective-C developers are also able to switch from iOS to OS X and vice-versa with very little difficulty. There are wrappers like
NW.js that let you build using HTML but it's not truly native and there will always be things you can't do.
At last year's WWDC, Apple introduced a new programming language that they see as the future of development on their platforms — Swift. Previously to build native apps for iOS and OS X (and now Apple Watch) you had to use Objective-C. I couldn't even get past the ugly syntax with Obj-C but Swift seemed to be much more friendly.
Learning Swift
I actually first wanted to learn Swift not for iOS but to build a Mac app. I watched a couple of tutorials and read through the iBook that Apple put out to get a feel for the language. The first thing that struck me was just how familiar it seemed. It's like a mash up of all the good from other languages with a couple of quirks of its own like Optionals and Extensions (Extensions are actually fucking awesome, more on them in a bit).
Once I was excited about diving into something new, I asked around for some recommendations on good resources. The best thing I found was the Cocoa Programming book from Big Nerd Ranch (they have one specifically for iOS too but I'd recommend learning OS X and then iOS as there are a few more complexities on the Mac).
While the language is simple enough, there are some new conventions and tools that you will need to learn if you are coming from the web.
Storyboards
Storyboards are just one of the ways you can build a user interface but if you're building for iOS or later versions of OS X it's probably what you should be using.
Storyboards allow you to layout your whole app visually and show the flow from one screen to another using segues. Segues can be automatically called by table rows or programmatically instigated. On both iOS and OS X there are different segue styles which control how the new view is presented on the screen. On iOS for example, these can be push, modal or a custom segue and on OS X you can open a new window or present as a sheet.
My main gripe with Storyboards is that they can get very very messy very very quickly. With iOS 9 and El Capitan Apple are fixing this by allowing placeholders to be added to a storyboard that link out to another. This will allow you to break up your app into multiple storyboards very easily. You can already have multiple storyboards but you're constantly having to load them manually and I couldn't be bothered with that.
What's fantastic about Storyboards on iOS is that they're universal and allow the adaptation of your UI at different size classes. Say you wanted to hide a specific element on iPhones but only in Landscape. Change the view class and then "uninstall" the element.
Auto Layout
Perhaps one of the best and most infuriating tools added in recent versions of iOS and OS X is Auto Layout. It works on the idea of constraints. Add constraints to specific elements to control margin, position on screen and size amongst other things.
It's something I'm yet to fully get to grips with and there are often yellow and red triangles alerting me that I'm a total idiot most times I try to do something remotely more advanced.
Thankfully, with iOS 9 Apple are adding Stack View which will do a lot of what we currently do in Auto Layout for us.
Constraints can also be added programmatically using the Visual Format Language which makes use of pipes and hyphens to replicate what you see when using Auto Layout in Interface Builder.
Protocols and Delegates
Protocols seem to be very similar to PHP's interfaces. If a class conforms to a Protocol it has access to methods defined by that protocol. The protocol can define both required and optional methods.
Delegates however were new to me. They're the main way that other classes fire notifications around. For example, a media player may have a delegate and send notifications to methods on your class to notify when the state has changed (play/pause) or when a file has finished loading.
There's a great talk about Protocol-Orientated Programming from this year's WWDC which really shows how powerful these things can be.
Assets
Assets (images) should be stored in an asset catalog which is essentially just a folder structure of image with a JSON file in there. I steered away from using any pixel based images to avoid the 1x, 2x and 3x nightmare.
Using PDFs is just easier and you can use these images as they are or render them as a template. Templates are able to be recoloured for your various states making it much easier than generating 6 images (3 for your "off" state, 3 for your "on" state).
Dependency Management
Like everything, you're gonna have dependencies in your projects. There are a couple of managers I found that will do a lot of the leg work for you: Cocoapods and Carthage.
Cocoapods does a lot more of the work for you by creating a workspace file. You don't need to import frameworks into your targets manually. Carthage on the other hand is completely decentralised and uses Github repos rather than a central directory like Cocoapods.
More info on the differences can be found on Carthage's repo.
Occasionally you may need to use some Objective-C libraries in your project. Swift lets you do this easily though the use of a Bridging Header.
Xcode
Putting all this aside though, perhaps the most important thing I had to learn when diving in is Xcode. It's a really powerful IDE but it can be quite intimidating when you first open it with all its drawers and tabs.
The Big Nerd Ranch book covers a lot of the basics of where things are and eventually you'll figure out where things are. Everything is in a pretty obvious place once you work out what all the various panels are for.
✌️
Overall I was completely surprised at how easy Swift and development for iOS and OS X was to pick up. While I haven't spoken about OS X's intricacies (cells, binding etc.), much of the principals are the same. Both use the same language, similar APIs, protocols & delegates etc. so it's easy to hop between the two.
I was able to learn the language and build my first app within 4 weeks. If you're wanting to get involved with native development and know some other language, just do it. Chances are Swift will feel very familiar and you'll pick it up easily.
== P.S. you should totally go download my first app Fetch ==